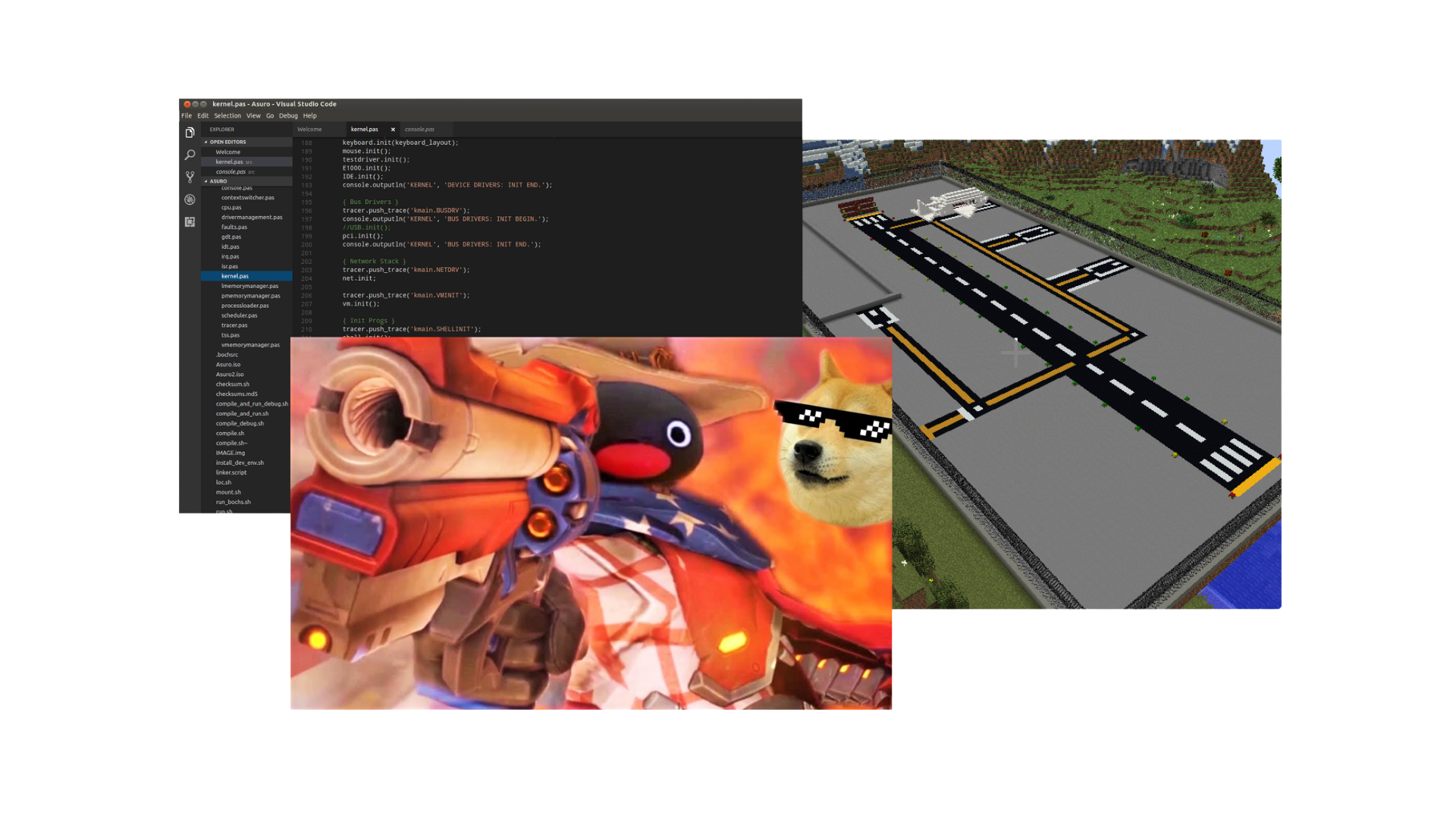
What is Spexeah?
Spexeah is a close-knit community of passionate developers, gamers, and tech enthusiasts who have been hanging out together since the TeamSpeak days. Whether you love coding, gaming, or just vibing with like-minded people, you'll feel at home here.
Why not Join Us?
- Active & Friendly Community - Chat, share memes, and engage in discussions on tech, gaming, and more.
- Collaborate on Projects - Join community-driven projects, find teammates, or get feedback on your own work.
- Regular Events & Game Nights - Compete, team up, and have fun in organized game nights and coding challenges.
- A Place to Learn & Grow - Whether you're a beginner or an expert, share knowledge and sharpen your skills.